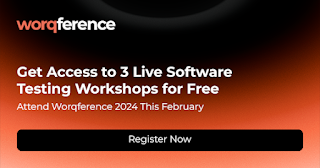
.
what is inheritance?
The process of acquiring properties (variables) &methods (behaviors) from one class to another class is called inheritance.
We are achieving inheritance concept by using extends keyword.
Inheritance Also known as is-a relationship.
Extends keyword is providing relationship between two classes..
The main objective of inheritance is code extensibility whenever we are extending the class automatically code is reused.
The process of acquiring properties (variables) &methods (behaviors) from one class to another class is called inheritance.
We are achieving inheritance concept by using extends keyword.
Inheritance Also known as is-a relationship.
Extends keyword is providing relationship between two classes..
The main objective of inheritance is code extensibility whenever we are extending the class automatically code is reused.
![]() |
Inheritance in java |
use of inheritance in java
Q:What is Use of inheritance in java ?
A: Inheritance use for
1)Stablish the Relationship between Parent and child class
2) To achieve the Method overriding
3) for code extensibility
Inheritance Example
Example without inheritance
class A
{
void m1(){ }
void m2(){ }
};
class B
{
void m1(){ }
void m2(){ }
void m3(){ }
void m4(){ }
};
a. Duplication of code.
b. Code length is increased.
Example with inheritance
class A //parent class or super class or base
{
void m1(){ }
void m2(){ }
};
class B extends A //child class or sub or derived
{
void m3(){ }
void m4(){ }
};
a. Eliminated duplication.
b. Length of the code is decreased.
c. Reusing properties in child classes.
Parent class or super class or base-
Child class or sub or derived-
extends keyword:-We are achieving inheritance concept by using extends keyword,Extends keyword is providing relationship between two classes..(parent and child )
Types of Inheritance in Java
There are Five types of inheritance in java
1)Single Inheritance
2)Multi level Inheritance
3)hierarchical Inheritance
4)Multiple Inheritance // not supported
5) Hybrid Inheritance // not supported
1)Single Inheritance
![]() |
Single Inheritance |
Single Inheritance Example
class Animal //Parent Class
{
void drink()
class Lion extends Animal // Child Class
class Test
void drink()
{
System.out.println("Animal Drinking");
}
}
}
class Lion extends Animal // Child Class
{
void bark()
void bark()
{
System.out.println("barking...");
}
}
}
class Test
{
public static void main(String args[])
public static void main(String args[])
{
Lion L=new Lion();
L.bark();
L.drink();
}
Lion L=new Lion();
L.bark();
L.drink();
}
}
2)Multi level Inheritance
Multi level Inheritance Example
class Animal //Parent Class
3)Hierarchical Inheritance
Hierarchical Inheritance Example
class Animal
2)Multi level Inheritance
![]() |
Multilevel Inheritance |
Multi level Inheritance Example
class Animal //Parent Class
{
void drink()
class Lion extends Animal // Child Class
void drink()
{
System.out.println("Animal Drinking");
}
}
}
class Lion extends Animal // Child Class
{
void realKing()
void realKing()
{
System.out.println("I am Real King ");
}
}
}
class Tiger extends Lion
{
void king()
void king()
{System.out.println("I am King ");
}
}
class Test
}
class Test
{
public static void main(String args[])
public static void main(String args[])
{
Tiger T =new Tiger();
T.king();
T.realKing();
Tiger T =new Tiger();
T.king();
T.realKing();
T.drink}
}
3)Hierarchical Inheritance
![]() |
Hierarchical Inheritance |
Hierarchical Inheritance Example
class Animal
{
void eat()
void eat()
{
System.out.println("Animal eating...");
}
}
class Lion extends Animal
}
class Lion extends Animal
{
void realKing()
void realKing()
{
System.out.println("I am Real King");
}
}
class Tiger extends Animal
}
class Tiger extends Animal
{
void King()
void King()
{
System.out.println("I am King.");
}
}
class Test
}
class Test
{
public static void main(String args[])
public static void main(String args[])
{
Tiger T=new Tiger();
T.king();
T.eat();
//T.realKing();//Compile Time Error
}
Tiger T=new Tiger();
T.king();
T.eat();
//T.realKing();//Compile Time Error
}
}
4)Multiple Inheritance // not supported
Multiple Inheritance Example
class X
4)Multiple Inheritance // not supported
![]() |
Multiple Inheritaqnce |
Multiple Inheritance Example
class X
{
void xyz()
void xyz()
{
System.out.println("India is great");}
}
class Y
}
class Y
{
void xyz()
void xyz()
{
System.out.println("India is nice");}
}
class Z extends X,Y
}
class Z extends X,Y
{//suppose if it were
public static void main(String args[])
public static void main(String args[])
{
Z Test=new Z();
Test .xyz();
}
}
//Compile time Error
5) Hybrid Inheritance // not supported
Hybrid Inheritance Example
Preventing Inheritance
Q: How to Prevent the inheritance in java ?
A: To prevent the inheritance follow the below step
You can prevent sub class creation by using final modifier.
If a class declared as final we can’t create sub class for that class.
final class A
{
}
class B extends A
{
}
compilation error:- cannot inherit from final Parent
Points to Remember about Inheritance
1)In java if we are extending the class then it will be parent class , if we are not extending the class then object class will become the default super class.
2)The root class of all java classes is “object” class.
3)Every java class contains parent class except object class.
4)In java every class is child class of object eitherdirectly (A) or indirectly (B,C).
5)Object class present in java.lang package and it contains 11 methods & all java classes able to use these 11 methods because Object class is root class of all java classes.
6)To check the predefined support use javap command.
syntax:Javap full-class-name
Tags: Java inheritance , Inheritance type ,Inheritance concept, inheritance ,
Related Post:
This article is contributed by Sandeep Yadav.
If you want to sahre your knowledge with us and would like to contribute, you can mail your article to qa.article@gmail.com.
Z Test=new Z();
Test .xyz();
}
}
//Compile time Error
5) Hybrid Inheritance // not supported
![]() |
Hybrid Inheritance |
Hybrid Inheritance Example
Preventing Inheritance
Q: How to Prevent the inheritance in java ?
A: To prevent the inheritance follow the below step
You can prevent sub class creation by using final modifier.
If a class declared as final we can’t create sub class for that class.
final class A
{
}
class B extends A
{
}
compilation error:- cannot inherit from final Parent
Points to Remember about Inheritance
1)In java if we are extending the class then it will be parent class , if we are not extending the class then object class will become the default super class.
2)The root class of all java classes is “object” class.
3)Every java class contains parent class except object class.
4)In java every class is child class of object eitherdirectly (A) or indirectly (B,C).
5)Object class present in java.lang package and it contains 11 methods & all java classes able to use these 11 methods because Object class is root class of all java classes.
6)To check the predefined support use javap command.
syntax:Javap full-class-name
Tags: Java inheritance , Inheritance type ,Inheritance concept, inheritance ,
Related Post:
This article is contributed by Sandeep Yadav.
If you want to sahre your knowledge with us and would like to contribute, you can mail your article to qa.article@gmail.com.
0 Comments