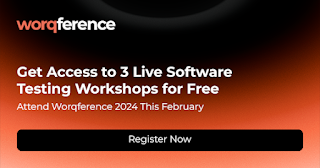
.
Handling Buttons in Selenium Webdriver
How To Click On Button In Selenium Webdriver Using Java?
To click on a button using Selenium webdriver we use the click() method. Before Clicking on the Button First, we have to identify the button to be clicked with the help of any locators like id, name, class, XPath, tagname, or CSS. Then we will apply the click() method.
Click(): Click method in selenium
In Selenium webdriver to click on any element, we use the click() method.
Selenium Codeimport org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class FacebookLogin{
public static void main(String[] args){
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver", "C:\\Software\\chromedriver.exe");
WebDriver driver=new ChromeDriver();
driver.get("https://www.facebook.com");
//Handling Multiple text Box in Selenium
//This is First Textbox
driver.findElement(By.xpath("//input[ @type=\"text\"]")).sendKeys("TextBox1");
//This is Second Textbox
driver.findElement(By.xpath("//input[@type=\"password\"]")).sendKeys("TextBox2");
//Click on buttondriver.findElement(By.id(""u_0_v"")).click;}
}
How to Get /Capture/ Retrieve Text of Button in Selenium Using Java?
To Capture the Button name in selenium just check out the below selenium code.
Selenium Codeimport org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class FacebookLogin{
public static void main(String[] args){
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver", "C:\\Software\\chromedriver.exe");
WebDriver driver=new ChromeDriver();
driver.get("https://www.facebook.com");
//Handling Multiple text Box in Selenium
//This is First Textbox
driver.findElement(By.xpath("//input[ @type=\"text\"]")).sendKeys("TextBox1");
//This is Second Textbox
driver.findElement(By.xpath("//input[@type=\"password\"]")).sendKeys("TextBox2");
//Click on the buttonString buttonname=driver.findElement(By.id(""u_0_v"")).getText();System.out.println(buttonname);
}}
How To Verify Text of Button in Selenium Using Java?
Selenium Codeimport org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class FacebookLogin{
public static void main(String[] args){
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver", "C:\\Software\\chromedriver.exe");
WebDriver driver=new ChromeDriver();
driver.get("https://www.facebook.com");
//Handling Multiple text Box in Selenium
//This is First Textbox
driver.findElement(By.xpath("//input[ @type=\"text\"]")).sendKeys("TextBox1");
//This is Second Textbox
driver.findElement(By.xpath("//input[@type=\"password\"]")).sendKeys("TextBox2");
//Click on the buttonString buttonname=driver.findElement(By.id(""u_0_v"")).getText();System.out.println(buttonname);
if (buttonname.contains("Log In"))
{
System.out.println("Valid Button name");
}
else
{
System.out.println("Invalid Button name");
}}
}
How To Check if Button is Disabled in Selenium Java?
isEnabled() Method :
In Selenium Webdriver isEnabled() method will verify and return a true value if the specified element is enabled. Else it will return a false value.
Selenium Codeimport org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class FacebookLogin{
public static void main(String[] args){
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver", "C:\\Software\\chromedriver.exe");
WebDriver driver=new ChromeDriver();
driver.get("https://www.facebook.com");
//Handling Multiple text Box in Selenium
//This is First Textbox
driver.findElement(By.xpath("//input[ @type=\"text\"]")).sendKeys("TextBox1");
//This is Second Textbox
driver.findElement(By.xpath("//input[@type=\"password\"]")).sendKeys("TextBox2");
//Click on the buttonBoolean nn=driver.findElement(By.id(""u_0_v"")).isEnabled();if(nn){
System.out.println("Yes ! Button is enable");
}
else{
System.out.println("No ! Button is not enable");
}
}}
Hope !!! The above tutorial on "How to Handle Buttons in Selenium web driver using Java" is helpful for you...
Team,
QA acharya
0 Comments