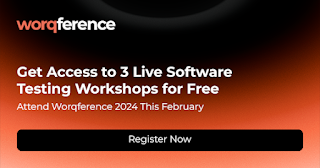
Handling Calendar in Selenium Webdriver Using Java
![]() |
How to handle Calendar in Selenium Webdriver |
How To Select a Date from Date Picker in Selenium Web Driver Using Java?
First Way:
Selenium Code:
Below the selenium code to handle the calendar is given..
public class CalendarinSelenium {
public static void main(String[] args)
{
// This line will Set the driver's path
System.setProperty("webdriver.firefox.marionette",
"G:\\Selenium\\Firefox driver\\geckodriver.exe");
// This line Will start firefox
WebDriver driver=new FirefoxDriver();
// This line will start application
driver.get("http://seleniumpractise.blogspot.com/2016/08/
how-to-handle-calendar-in-selenium.html");
// This line will click on the date picker so that we can get the calendar in view
driver.findElement(By.id("datepicker")).click();
// This line will find all matching nodes in the calendar
List<WebElement> allDates=driver.findElements(By.xpath
("//table[@class='ui-datepicker-calendar']//td"));
// This line will iterate all values and will capture the text. We will select the date is 28
for(WebElement ele:allDates)
{
String date=ele.getText();
// once date is 28 then click and break
if(date.equalsIgnoreCase("28"))
{
ele.click();
break;
}
}
}
}
Second Way :
Selenium Code :
Below Selenium Code will handle the Calendar using CSS and Xpath.
public class HandlingCalendarinSelenium {
public static void main(String[] args)
{
// This Line will Set the driver path
System.setProperty("webdriver.firefox.marionette","G:\\Selenium\\Firefox driver\\geckodriver.exe");
// This Line will start Firefox
WebDriver driver=new FirefoxDriver();
// This Line will start application
driver.get("http://seleniumpractise.blogspot.com/2016/08/how-to-handle-calendar-in-selenium.html");
// This Line will click on the date picker so that we can get the calendar in view
driver.findElement(By.id("datepicker")).click();
// This Line will find all matching nodes in the calendar
List<WebElement> allDates=driver.findElements(By.xpath("//table[@class='ui-datepicker-calendar']//td"));
// Click on date 28 or any other date of your choice
driver.findElement(By.xpath("//a[text()='28']")).click();
}
}
In selenium to click on a calendar we use the click method.
public class CalendarDateField {
public static void main(String[] args) throws InterruptedException {
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver",
"C:\\Users\\Admin\\Downloads\\chromedriver-win64 (1)\\chromedriver-win64\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("http://seleniumpractise.blogspot.com/2016/08/
how-to-handle-calendar-in-selenium.html");
// click on date picker so that we can get the calendar in view
driver.findElement(By.id("datepicker")).click();
}
}
Selecting Future dates, Current dates and Past dates from Date Picker in Selenium Webdriver Using Java.
In this section, we are going to learn how to select a future date from the date picker in the Selenium web driver using Java.
Selenium Code :
Below the selenium code to select the future date from the date picker is given.
public class CalendarDateField {
public static void main(String[] args) throws InterruptedException {
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver"
,"C:\\Users\\Admin\\Downloads\\chromedriver-win64 (1)\\chromedriver-win64\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("http://seleniumpractise.blogspot.com/2016/08/
how-to-handle-calendar-in-selenium.html");
// click on date picker so that we can get the calendar in view
driver.findElement(By.id("datepicker")).click();
Thread.sleep(1000);
while (true)
{
String selmnth=driver.findElement(By.className("ui-datepicker-title")).getText();
//String selyer=driver.findElement(By.className("ui-datepicker-year")).getText();
if (selmnth.equals("May 2024"))
{
break;
}
else {
driver.findElement(By.xpath("//a[@data-handler=\"next\"]")).click();
}
}
driver.findElement(By.xpath("//td[@data-handler=\"selectDay\"] /a[text()='21']")).click();
}
}
How to Select Current Date From Date Picker in Selenium web driver using Java?
In this section, we are going to learn how to select a Current date from the date picker in the Selenium web driver using Java.
Selenium Code :
Below the selenium code to select the Current date from the date picker is given.
In this section, we are going to learn how to select a Past date from the date picker in the Selenium web driver using Java.
Selenium Code :
Below the selenium code to select the Pasr date from the date picker is given.
public class CalendarDateField {
public static void main(String[] args) throws InterruptedException {
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver"
,"C:\\Users\\Admin\\Downloads\\chromedriver-win64 (1)\\chromedriver-win64\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("http://seleniumpractise.blogspot.com/2016/08/
how-to-handle-calendar-in-selenium.html");
// click on date picker so that we can get the calendar in view
driver.findElement(By.id("datepicker")).click();
Thread.sleep(1000);
while (true)
{
String selmnth=driver.findElement(By.className("ui-datepicker-title")).getText();
//String selyer=driver.findElement(By.className("ui-datepicker-year")).getText();
if (selmnth.equals("May 2020"))
{
break;
}
else {
driver.findElement(By.xpath("//a[@data-handler=\"prev\"]")).click();
}
}
driver.findElement(By.xpath("//td[@data-handler=\"selectDay\"] /a[text()='21']")).click();
}
}
Explanation: The above selenium Code will Select the date "21 May 2020" From the Date Picker as we have passed 21 May 2020 as a Past Date.
In this section, we are going to learn how to select a Custom date from the date picker in the Selenium web driver using Java.
Selenium Code :
Below the selenium code to select the Custom date from the date picker is given.
public class CalendarDateField {
public static void main(String[] args) throws InterruptedException {
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver"
,"C:\\Users\\Admin\\Downloads\\chromedriver-win64 (1)\\chromedriver-win64\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("http://seleniumpractise.blogspot.com/2016/08/
how-to-handle-calendar-in-selenium.html");
// click on date picker so that we can get the calendar in view
driver.findElement(By.id("datepicker")).click();
Thread.sleep(1000);
while (true)
{
String selmnth=driver.findElement(By.className("ui-datepicker-title")).getText();
//String selyer=driver.findElement(By.className("ui-datepicker-year")).getText();
if (selmnth.equals("May 2020"))
{
break;
}
else {
driver.findElement(By.xpath("//a[@data-handler=\"prev\"]")).click();
}
}
driver.findElement(By.xpath("//td[@data-handler=\"selectDay\"] /a[text()='21']")).click();
}
}
Explanation: The above selenium Code will Select the date "21 May 2020" From the Date Picker as we have passed 21 May 2020 as a custom Date.
Selenium Code :
public class DateField {
public static void main(String[] args) throws InterruptedException {
// TODO Auto-generated method stub
System.setProperty("webdriver.chrome.driver","C:\\Users\\Admin\\Downloads\\chromedriver-win64 (1)\\chromedriver-win64\\chromedriver.exe");
WebDriver driver = new ChromeDriver();
driver.get("https://seleniumpractise.blogspot.com/2016/08/how-to-handle-calendar-in-selenium.html");
WebElement ele=driver.findElement(By.id("datepicker"));
ele.sendKeys(" 05/01/2020");
Thread.sleep(5000);
ele.clear();
ele.sendKeys("06/10/2023");
}
}
Hope!!! The above tutorial on "Handling Date Picker in Selenium Using Java "Is helpful for you...
Team,
QA acharya
0 Comments